Graph vs. Tree
What's the Difference?
Graphs and trees are both data structures used to represent relationships between objects. However, there are some key differences between the two. A graph is a collection of nodes or vertices connected by edges, where each edge can have a weight or a direction. It allows for more complex relationships and can have cycles. On the other hand, a tree is a special type of graph that does not contain any cycles and has a hierarchical structure. It consists of nodes connected by edges, with a single root node and each node having a parent-child relationship. Trees are commonly used for organizing data in a hierarchical manner, while graphs are more versatile and can represent a wider range of relationships.
Comparison
Attribute | Graph | Tree |
---|---|---|
Definition | A collection of nodes (vertices) connected by edges | A hierarchical structure with a root node and child nodes |
Acyclic | May or may not be acyclic | Always acyclic |
Connectivity | Can be connected or disconnected | Always connected |
Direction | Edges can be directed or undirected | Edges are always directed from parent to child |
Root | No root node | Has a single root node |
Cycles | Can have cycles | No cycles |
Branching Factor | No specific branching factor | Each node can have multiple child nodes |
Traversal | Depth-First Search (DFS) or Breadth-First Search (BFS) | Pre-order, In-order, Post-order traversal |
Representation | Adjacency Matrix, Adjacency List | Parent-Child Relationship, Linked List |
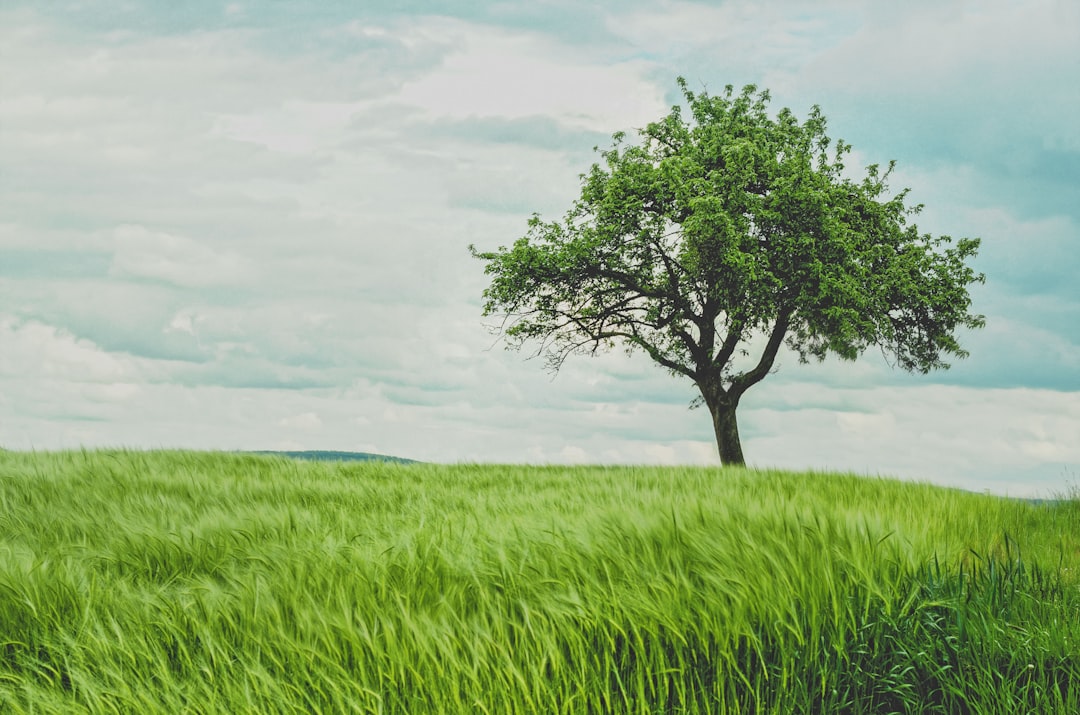
Further Detail
Introduction
Graphs and trees are fundamental data structures used in computer science and mathematics. While they share some similarities, they also have distinct characteristics that make them suitable for different applications. In this article, we will explore the attributes of graphs and trees, highlighting their similarities and differences.
Definition and Structure
A graph is a collection of nodes (also known as vertices) connected by edges. The edges can be directed or undirected, representing the relationships between the nodes. On the other hand, a tree is a type of graph that is acyclic, meaning it does not contain any cycles or loops. In a tree, there is a unique node called the root, and every other node is connected to it through edges. Each node in a tree can have zero or more child nodes, forming a hierarchical structure.
Connectivity
In terms of connectivity, both graphs and trees can be connected or disconnected. A connected graph is one where there is a path between any two nodes, while a disconnected graph has one or more isolated components. Similarly, a connected tree is one where every node is reachable from the root, while a disconnected tree would have one or more subtrees that are not connected to the root.
Representation
Graphs can be represented in various ways, including adjacency matrix, adjacency list, and edge list. The adjacency matrix is a two-dimensional array where each cell represents the presence or absence of an edge between two nodes. An adjacency list, on the other hand, is a collection of linked lists or arrays, where each node is associated with a list of its neighboring nodes. An edge list simply lists all the edges in the graph.
Trees, being a special type of graph, can also be represented using similar data structures. One common representation is the parent array, where each node stores the index of its parent node. Another representation is the child-sibling representation, where each node has a pointer to its first child and a pointer to its next sibling.
Traversal
Both graphs and trees can be traversed using various algorithms. One common algorithm for graph traversal is Depth-First Search (DFS), where we explore as far as possible along each branch before backtracking. Another algorithm is Breadth-First Search (BFS), which explores all the neighbors of a node before moving on to the next level.
For trees, there are additional traversal algorithms specific to their hierarchical structure. Pre-order traversal visits the root node first, followed by its left subtree, and then its right subtree. In-order traversal visits the left subtree, then the root node, and finally the right subtree. Post-order traversal visits the left subtree, then the right subtree, and finally the root node.
Applications
Graphs are widely used in various applications, including social networks, transportation networks, and computer networks. They can represent relationships between individuals, cities, or devices, allowing for efficient analysis and optimization. Graph algorithms such as Dijkstra's algorithm and the Minimum Spanning Tree algorithm are essential in solving real-world problems.
Trees, on the other hand, are commonly used in hierarchical structures such as file systems, organization charts, and decision trees. They provide a natural way to represent parent-child relationships and are efficient for searching, sorting, and organizing data. Binary search trees, AVL trees, and B-trees are examples of tree structures used in database systems and file systems.
Complexity Analysis
When it comes to complexity analysis, both graphs and trees have different characteristics. The number of edges in a graph can vary greatly, affecting the time and space complexity of graph algorithms. In the worst case, a graph with n nodes can have up to n*(n-1)/2 edges, resulting in quadratic time complexity for certain operations.
Trees, on the other hand, have a more predictable structure. The height of a tree, which is the maximum number of edges from the root to a leaf, determines the time complexity of tree operations. Balanced trees, such as AVL trees and Red-Black trees, ensure that the height remains logarithmic, resulting in efficient operations with time complexity of O(log n).
Conclusion
In conclusion, graphs and trees are both important data structures with their own unique attributes. While graphs are more general and versatile, allowing for complex relationships and connectivity, trees provide a hierarchical structure that is efficient for organizing and searching data. Understanding the characteristics and applications of graphs and trees is crucial for designing efficient algorithms and solving real-world problems.
Comparisons may contain inaccurate information about people, places, or facts. Please report any issues.